Firebase Push notifications using Admin SDK
July 1, 2021 by Swapnil Bhojwani
How to send push notifications through an Android app using firebase?
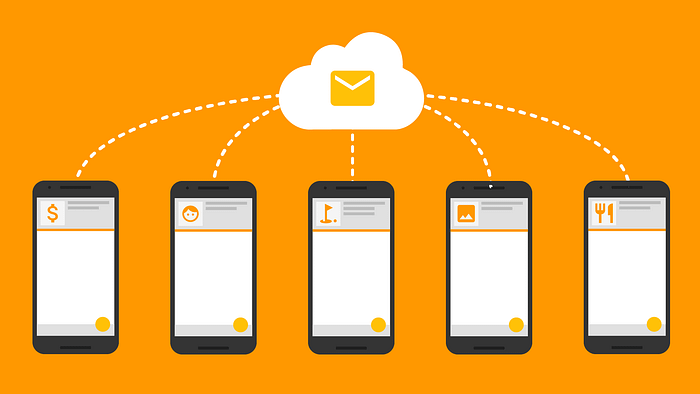
A notification is a message you can display to the user outside of your application’s normal UI. When you tell the system to issue a notification, it first appears as an icon in the notification area. Notifications are important to give timely updates to your android app users they require minimal UI to notify the user about the event.
Why Firebase Cloud Messaging (FCM) ?
Firebase Cloud Messaging (FCM) is a cross-platform messaging solution that lets you reliably send messages at no cost. Using FCM, you can notify a client app that new email or other data is available to sync. You can send notification messages to drive user re-engagement and retention. For use cases such as instant messaging, a message can transfer a payload of up to 4000 bytes to a client app.
Let’s dive into it’s code
Step 1:
Connect your android app to firebase.
Step 2:
Now add dependencies as given in app level build.gradle file 👇👇.
dependencies {
//Firebase BOM
implementation platform('com.google.firebase:firebase-bom:28.2.0')
//Messaging dependency
implementation 'com.google.firebase:firebase-messaging'
// define a BOM and its version
implementation(platform("com.squareup.okhttp3:okhttp-bom:4.9.1"))
// define any required OkHttp artifacts without version
implementation("com.squareup.okhttp3:okhttp")
}
Step 3:
Now make a java class named as FCMReceiver.java this class extends FirebaseMessagingService and it’s overriden method onMessageRecieved is called automatically called when a message comes into the app when the app is whether foreground or background. Here is the FCMReceiver code 👇👇.
public class FCMReceiver extends FirebaseMessagingService {
private static final String CHANNEL_ID = "Notification_channel";
Random random = new Random();
/*
* This is automatically called when notification is being received
* */
@Override
public void onMessageReceived(@NonNull @NotNull RemoteMessage remoteMessage) {
super.onMessageReceived(remoteMessage);
createNotificationChannel();
if (remoteMessage.getData().get("for") != null) {
if (remoteMessage.getData().get("for").equals(FirebaseAuth.getInstance().getCurrentUser().getPhoneNumber())) {
showNotification(remoteMessage);
}
}
}
/*
* Method to show notification when received
* */
private void showNotification(RemoteMessage remoteMessage) {
NotificationCompat.Builder builder = new NotificationCompat.Builder(this, CHANNEL_ID)
.setContentTitle(getString(R.string.app_name))
.setSmallIcon(R.drawable.ic_notification_icon)
.setContentText(remoteMessage.getData().get("body"))
.setColor(ContextCompat.getColor(this,R.color.notification_red));
NotificationManagerCompat notificationManager = NotificationManagerCompat.from(this);
// notificationId is a unique int for each notification that you must define
notificationManager.notify(random.nextInt() + 1000, builder.build());
}
/*
* Method to create notification channel
* */
private void createNotificationChannel() {
// Create the NotificationChannel, but only on API 26+ because
// the NotificationChannel class is new and not in the support library
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
CharSequence name = getString(R.string.channel_name);
String description = getString(R.string.channel_description);
int importance = NotificationManager.IMPORTANCE_DEFAULT;
NotificationChannel channel = new NotificationChannel(CHANNEL_ID, name, importance);
channel.setDescription(description);
NotificationManager notificationManager = getSystemService(NotificationManager.class);
notificationManager.createNotificationChannel(channel);
}
}
}
Step 4:
Now declare this code into AndroidManifest.xml as given in the code snippet👇👇.
<service
android:name=".notifications.FCMReceiver"
android:exported="false">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT" />
</intent-filter>
</service>
Step 5:
- Now our FCMReceiver.java is ready now we will move forward to see FCMSender code will we send notification by using the following code 👇👇.
package com.swapnil.helloworld.notifications;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.MediaType;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
public class FCMSender {
/*
* URL where we request to send notification and the key to send notification using admin sdk
* */
private static final String FCM_URL = "https://fcm.googleapis.com/fcm/send", KEY_STRING = "key=YOUR_ADMIN_SDK_KEY";
OkHttpClient client = new OkHttpClient();
/*
* Method to send notification to the application
* */
public void send(String message, Callback callback) {
RequestBody reqBody = RequestBody.create(message
, MediaType.get("application/json"));
Request request = new Request.Builder()
.url(FCM_URL)
.addHeader("Content-Type", "application/json")
.addHeader("Authorization", KEY_STRING)
.post(reqBody)
.build();
Call call = client.newCall(request);
call.enqueue(callback);
}
}
- YOUR_ADMIN_SDK_KEY will be replaced by the key given in your firebase project it is located in tab of cloud messaging under project settings see the below screenshot to locate the key
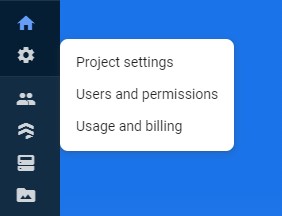

NOTE:- Don’t share the key with anyone else otherwise there will be breach in your application someone having this key can send push notifications to your application so beware.
TIP:- If Breach occurs then click on add server key and delete the older key.
Step 6:
Call the FCMSender.send() method to send notification to the application and you have to give topic to which you wanna send message. If the user is subscribed to the topic then he/she will get notification message. Here we have to pass topic to which we want to send notification. And in “for” we have to send mobile number with country code appended in it, and in “body” you can pass the message you want to send. The message you have to pass while sending notification have format as that of the message string given below 👇👇.
package com.swapnil.helloworld.notifications;
public class NotificationMessage {
public static String message = "{" +
" \"to\": \"/topics/%s\"," +
" \"data\": {" +
" \"body\":\"%s\"," +
" \"for\":\"%s\""+
" }" +
"}";
}
Data vs Notification
We have used data in the message because there is reason behind it we also want to show notifications when the application is in background so we have used data but if you have sent notification instead it does not execute the code written in onMessageRecieved so we cannot customize it and it also has one disadvantage it is shown by system so it is shown only when the app is in background i.e app must be in recents otherwise notification will be killed.
Code sample for using Notification as message 👇👇.
package com.swapnil.helloworld.notifications;
public class NotificationMessage {
public static String message = "{" +
" \"to\": \"/topics/%s\"," +
" \"notification\": {" +
" \"body\":\"%s\"," +
" \"title\":\"%s\""+
" }" +
"}";
}
[Here is the youtube video of the application that i have made for the blog](Android app to send notification using FCM and Admin SDK Firebase - YouTube)
For more stuff related to programming follow me. Here is the source code to this application GitHub - swapnil20711/NotificationsAdminSDK
Happy coding🔥🔥😀😀.