Implement Notification Count overlay inside Android App
May 20, 2021 by Atul Sharma

Do you want to add notification count or badge like this to your app? Well everyone developer wants to add it as it improves User Experience significantly.
Its always handy to know how many notification I have when I open my app.
For the demo purpose we shall be creating this layout.
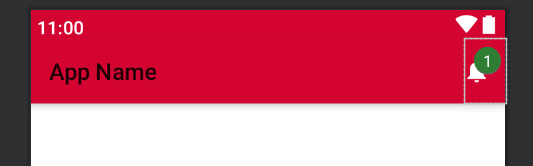
Step 1
Add this custom UI implementation to app-level gradle
file. We will be using this library.
implementation 'com.nex3z:notification-badge:1.0.4'
Step 2
Create a layout for the icon res/layout/notification_icon_overlay.xml
.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto">
<ImageView
android:id="@+id/image"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:src="@drawable/ic_baseline_notifications_24" /> // icon for image
<com.nex3z.notificationbadge.NotificationBadge
android:id="@+id/badge_count"
android:layout_toEndOf="@id/image"
android:layout_alignTop="@id/image"
android:layout_marginStart="-18dp"
app:nbAnimationEnabled="true"
app:nbAnimationDuration="1000"
android:layout_marginTop="4dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:nbBackground="@drawable/notification_badge"
app:nbMaxTextLength="3"/>
</RelativeLayout>
Step 3
Create a menu item to show that layout in app bar res/menu/notification_menu.xml
. Add the above layout inside this item under actionLayout
attribute.
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<item
android:title="@string/notification"
android:icon="@drawable/ic_baseline_notifications_24"
android:id="@+id/notification_bell"
android:actionLayout="@layout/notification_icon_overlay"
app:showAsAction="ifRoom"/>
</menu>
Now you should be able to see its preview in Studio.
Step 4
Inflate this menu item in our MainActivity by overriding the onCreateOptionsMenu
method.
static NotificationBadge mBadge;
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.notifications_menu, menu);
// getting item id to get its layout
MenuItem item = menu.findItem(R.id.notification_bell);
MenuItemCompat.setActionView(item, R.layout.notification_icon_overlay);
// getting the layout from menu option to set badge actions
RelativeLayout notifCount = (RelativeLayout) MenuItemCompat.getActionView(item);
notifCount.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
openNotificationActivity(); // what happens when icon is clicked
}
});
mBadge = notifCount.findViewById(R.id.badge_count);
mBadge.setNumber(getNotificationsCount());
return true;
}
Here getNotificationCount()
method returns the integer about how many notification your app may have. If you set 0 (zero) on setNumber
method the overlay will not display.
Step 5
If you wish to update notification counter after the activity is created or after opening the notifications activity call this method.
@Override
protected void onResume() {
super.onResume();
invalidateOptionsMenu(); // it will make all the menu items to be redrawn.
}
invalidateOptionsMenu()
can be called only from activities life cycle methods. Placing it somewhere else won’t work.
That’s It 🙌. In just 5 steps you created a notification badge overlay.
Hope it helped. Happy Coding 😀