Implementing the Perfect Splash for Android
May 15, 2021 by Atul Sharma
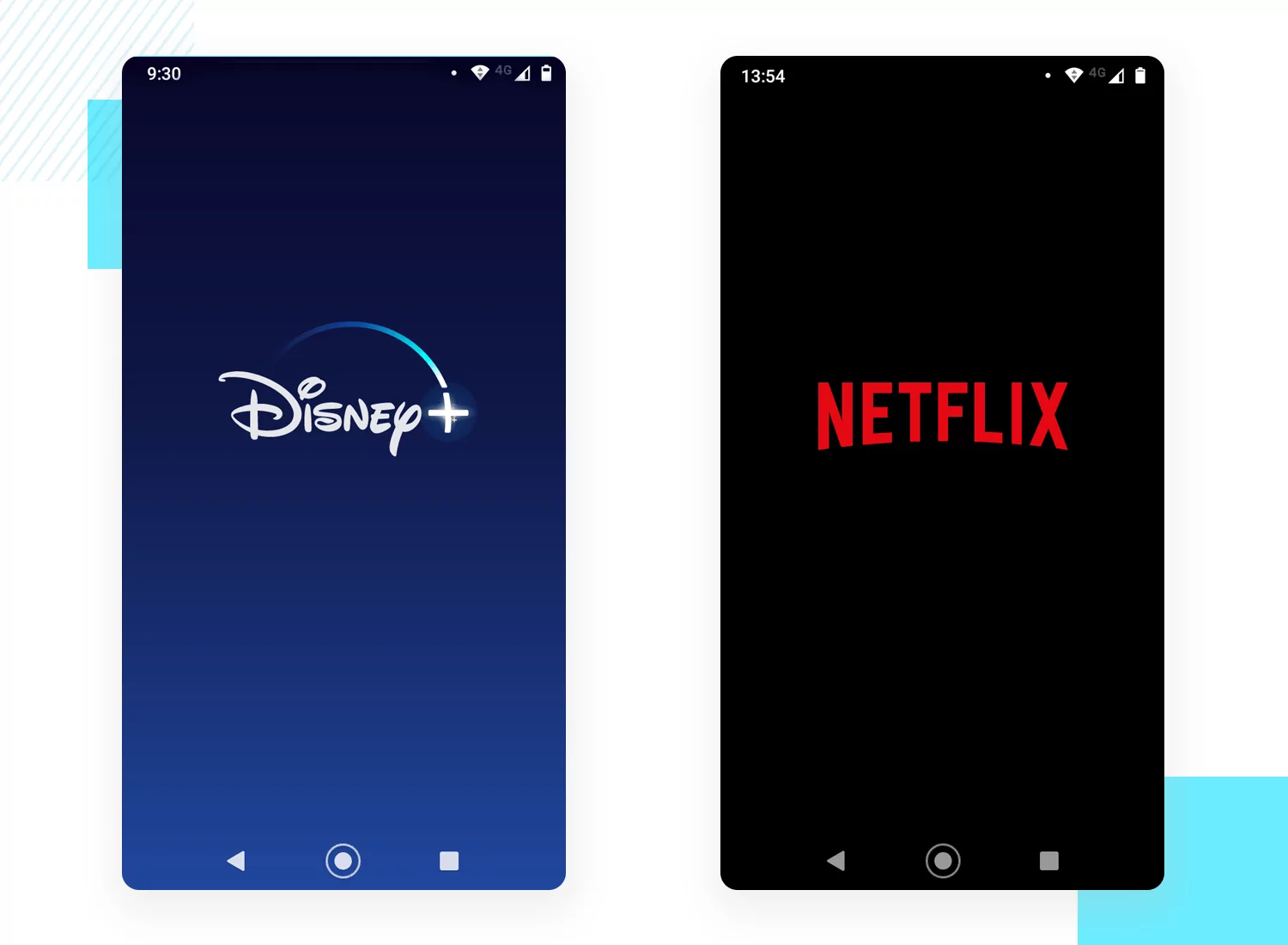
A Splash Screen is a screen which appears when you open an app on your mobile device. Sometimes it’s referred to as a launch screen or startup screen and shows up when your app is loading after you’ve just opened it.
When the loading is finished, you’ll be taken to a more functional screen where you can complete actions.
Splash screens appear on your screen for a fleeting moment – look away and you might miss them. Splash screen is one of the most vital screens in the application since it’s the user’s first experience with the application.
There are 2 common methods of implementing splash screens and will find the right way:
- Using Timers (the bad)
- Using a Launcher Theme (The Right way)
Using timer ( Our 1st Method ) into your activity to display splash, we create a splash activity and create a thread in onCreate()
for shows up for 2/3 seconds, then go to our desired activity. Here see the implementation of this easy method:
public class SplashScreenActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_splash_screen);
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
// This method will be executed once the timer is over
// Start your app main activity
Intent i = new Intent(SplashScreenActivity.this, MainActivity.class);
startActivity(i);
// close this activity
finish();
}
}, 3000);
}
}
The above approach isn’t the correct approach. It’ll give rise to cold starts.
The purpose of a Splash Screen is to quickly display a beautiful screen while the application fetches the relevant content if any (from network calls/database).With the above approach, there’s an additional overhead that the SplashActivity
uses to create its layout.
It’ll give rise to slow starts to the application which is bad for the user experience (wherein a blank black/white screen appears).
The cold start appears since the application takes time to load the layout file of the Splash Activity. So instead of creating the layout, we’ll use the power of the application theme to create our initial layout (Our 2nd Method).
Application theme
is instantiated before the layout is created. We’ll set a drawable inside the theme that’ll comprise of the Activity’s background and an icon using layer-list as shown below.
Do you know when an activity is called Android OS first see in the manifest is there any theme for that activity and load the theme from manifest?
So we will set a custom theme in Manifest for our splash activity. To create the theme for splash screen follow the below process.
- Create Background for Splash Screen in
drawable/splash_background.xml
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:drawable="@drawable/bg_gradient"/>
<item>
<bitmap android:gravity="center"
android:src="@drawable/splash_fg"/>
</item>
</layer-list>
- Create the gradient onto which your app logo will be placed in
drawable/bg_gradient.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle" >
<gradient
android:type="linear"
android:angle="135"
android:startColor="#ff1b49"
android:endColor="#e67d20" />
</shape>
- Create Style for Splash Screen in
res/values/themes.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<style name="SplashTheme" parent="Theme.MaterialComponents.Light.NoActionBar">
<item name="android:windowBackground">@drawable/splash_background</item>
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
<item name="android:statusBarColor">@color/colorAccent</item>
</style>
</resources>
- Set the style as theme for SplashScreenActivity in
AndroidManifest.xml
<activity android:name=".SplashScreenActivity"
android:theme="@style/SplashTheme">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>.
- Setting the Intent to your MainActivity.java from
SplashScreenActivity.java
public class SplashScreenActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
startActivity(new Intent(SplashScreenActivity.this, MainActivity.class));
finish();
}
}
**As we are loading the splash screen them from manifest, there is no need to
setContentView
() with any **xml layout. If you want to show your splash screen for a some amount of time (like 5 secs) you can create aHandler
.
Using the theme and removing the layout from the SplashScreenActivity
is the correct way to create a splash screen 🙌**.
Now that our splash is working, what size of image should I put into drawable folder?
Always put your app_logo.png
into drawable-xxhdpi
folder so that your logo automatically scales for all types of phone screens in most cases.
Also make sure that image resolution is not more than 1000x1000 pixels
. It can have less resolution depending upon how you want it.
Now there are different types of splash screen which has multiple logo on different parts of screen. How that could be done?
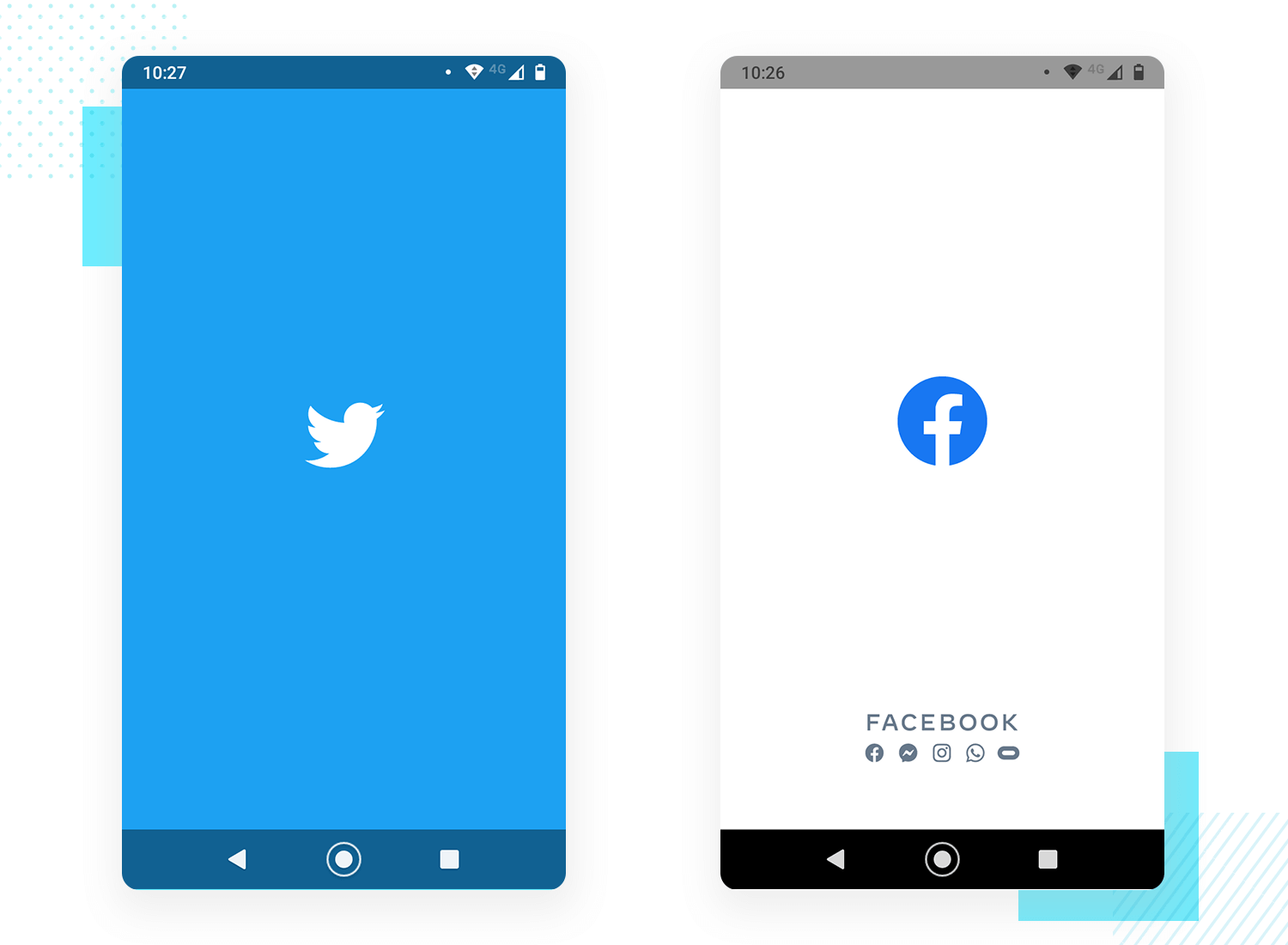
From above example, we implemented our method to create splash like Twitter and now to create one like Facebook we just have to make a small change to our drawable/splash_background.xml
.
Just add as many item
to your layer-list
you want to place in your splash screen.
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/bg_gradient"/>
<item>
<bitmap android:gravity="center"
android:src="@drawable/logo_art_1"/>
</item>
<item android:bottom="50dp"> // setting the image from bottom
<bitmap android:gravity="bottom"
android:src="@drawable/logo_art_2"/>
</item>
</layer-list>
Splash screen best practices
Splash screens are simple. They’re used to enhance a brand and give users something nice to look at as they wait. In this regard, here are some best practices for when you design your own splash screen:
- Keep it free from unnecessary distraction
- Don’t use multiple colors or logos
- Use animation sparingly
Hope it helped. Happy Coding 😀